Front-end applications must communicate with a server using the HTTP protocol, to upload and download the data as well as other backend services. And angular does this very efficiently. The improved features of Angular 11 enables us to seamlessly communicate with different devices through API interfaces. Let’s understand how API calls can be made using Angular.
Angular HttpClient Module:
Angular is an open-source platform that makes it easy to create applications within web/mobile/desktop. As such, you are not required to use other external libraries to carry out common operations such as HTTP requests. Angular provides the HttpClient module which enables developers to send HTTP requests and execute API calls to remote HTTP servers. You can import the HttpClient module from the @angular/http library and it replaces the previous http client which was available from the @angular/http library.
- In web browsers, there are two standard APIs to send HTTP requests, the fetch () API and the XMLHttpRequest.
- The HttpClient module is constructed on the XMLHttpRequest interface. It envelops all the complexities of the interface and provides additional functionality such as:
- Better error handling
- Supporting for testing
- Requests and responses types
- RxJS observables
- Interceptors for requests
Configuring the HttpClient Module:
After the introduction of HttpClient, let’s see how it can be configured in the Angular Application. First, you need to import the HttpClient module from the @angular/common/http library and include this in the imports array.
import { HttpClientModule } from ‘@angular/common/http’;
You can include the HttpClient module in the imports array like
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
HttpClientModule
],
In the app.module.ts file, import the HttpClient module.
import { BrowserModule } from ‘@angular/platform-browser’;
import { NgModule } from ‘@angular/core’;
import { AppRoutingModule } from ‘./app-routing.module’;
import { AppComponent } from ‘./app.component’;
import { FormsModule } from ‘@angular/forms’;
import { HttpClientModule } from ‘@angular/common/http’;
@NgModule({
declarations: [
AppComponent,
ChildComponent
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule,
HttpClientModule
],
providers: [],
bootstrap: [ AppComponent ]
})
export class AppModule { }
After that, you can use the HttpClient module to send the requests to an API. In the next step, you will create a service that encapsulates the code to communicate the server.
Creating an Angular Service:
After configuring the HttpClient module in the project, you need to create a service that will import the HttpClient and use it to send the Http requests required for your application. These services will be injected into any components which require to do HTTP operations.
You can generate the services by the following command.
ng g s Demoapi
You need to create a class that will be used as the type of every retrieved object. You can generate the class using the following command.
ng g class employee
This command can generate the model file. In the employee.ts file, add the following code.
export class Employee {
id: number;
firstName: string;
lastName: string;
email: string;
phone: number;
city: string;
}
Next, import the HttpClient in the demoapi.service.ts file.
import { Injectable } from ‘@angular/core’;
import { HttpClient } from ‘@angular/common/http’;
@Injectable({
providedIn: ‘root’
})
export class DemoapiService {
APP_URL: ‘http://192.168.0.88:8098/api’
constructor(private httpClient: HttpClient) { }
}
First, you inject the HttpClient as a private httpClient instance into the constructor. After that add the APP_URL variable that stores the address of the API server. Next, you will add the methods of reading, create, update and delete operations.
After creating the class modal and service, you can create the crud methods. These methods will use the employee modal class as a type. You need to first import the employee class in the service file.
import { Employee } from ‘./employee’;
Then, you need to define the following methods for reading, creating, updating, and deleting the employee.
public createEmployee (employee: Employee) { }
public updateEmployee (employee: Employee) { }
public deleteEmployee (id: number) { }
public getEmployeeById (id: number) { }
public getEmployee () {}
– The createEmployee() method takes a parameter of the Employee type and creates a new employee.
– The updateEmployee() method updates the Employee.
– The deleteEmployee() method deletes an employee by id.
– The getEmployee() method get all the employees.
– The getEmployeeById() method gets the employee by id.
After that, you will implement all the methods one by one in beginning with the getEmployee() method.
Fetching the Employee List:
public getEmployee() {
return this.httpClient.get<Employee []> (`${this.APP_URL}/employees`);
}
This will return an Observable<Employee []> which you must subscribe to in the components, to retrieve the employee data from the server.
Fetching the single object by its Identifier:
public getEmployeeById(id: number) {
return this.httpClient.get(`${this.APP_URL}/employees/${id}`);
}
In this method, you can get a single employee by id.
Sending post request:
You can add the createEmployee() method which sends a post request into the server. It uses a parameter of type Employee.
public createEmployee(employee: Employee){
return this.httpClient.post(`${this.APP_URL}/employees/`, employee);
}
Sending put request:
After creating the method of the createEmployee(), you will create the updateEmployee() method which will be used to update the employee data.
public updateEmployee(employee: Employee) {
return this.httpClient.put(`${this.APP_URL}/employees/${employee.id}`,employee);
}
Sending delete request:
The deleteEmployee() method deletes the employee by its identifier.
public deleteEmployee(id: number){
return this.httpClient.delete(`${this.APP_URL}/employees/${id}`);
}
Adding headers:
Most Http requests includes headers. The Http Headers enable the client and server to share the extra information regarding the Http request or response. For example, we can use the content-type header to specify the media type of the resource such as text, JSON, etc. Another important header is to send the bearer token through the authorization header ’Authorization’, ‘Bearer yourToken’.
To use the HttpHeaders within your application, you need to import it into your service. You can add the headers from the ‘@angular/common/http’ library.
import { HttpHeaders } from ‘@angular/common/http’;
Add a constant variable to defines the HttpHeaders parameters.
const httpOptions = {
headers: new HttpHeaders({
‘Content-Type’: ‘application/json’
})
}
In the demoapi.service.ts file, add the headers and implement the methods.
import { Injectable } from ‘@angular/core’;
import { HttpClient, HttpHeaders } from ‘@angular/common/http’;
import { Employee } from ‘./employee’;
@Injectable({
providedIn: ‘root’
})
export class DemoapiService {
APP_URL: ‘http://192.168.0.88:8098/api’;
constructor( private httpClient: HttpClient ) { }
const httpOptions = {
headers: new HttpHeaders({
‘Content-Type’: ‘application/json’
})
}
public getEmployee() {
return this.httpClient.get<Employee[]>(`${this.APP_URL}/employees`);
}
public getEmployeeById(id: number) {
return this.httpClient.get(`${this.APP_URL}/employees/${id}`);
}
public createEmployee(employee: Employee) {
return this.httpClient.post(`${this.APP_URL}/employees/`,employee);
}
public updateEmployee(employee: Employee) {
return this.httpClient.put(`${this.APP_URL}/employees/${employee.id}`,employee);
}
public deleteEmployee(id: number) {
return this.httpClient.delete(`${this.APP_URL}/employees/${id}`);
}
}
Conclusion:
In this blog, we have seen what is HttpClient module, the functionalities of the module, and how to configure this module in Angular Application.
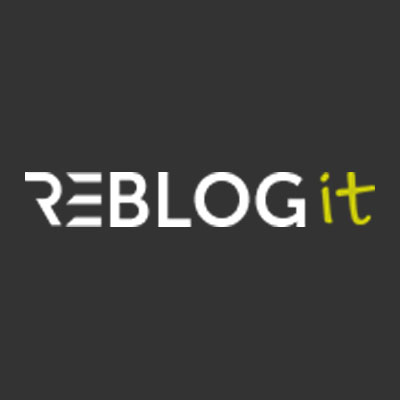
Reblog It collaborates closely with clients to develop tailored guest posting strategies that align with their unique goals and target audiences. Their commitment to delivering high-quality, niche-specific content ensures that each guest post not only meets but exceeds the expectations of both clients and the hosting platforms. Connect with us on social media for the latest updates on guest posting trends, outreach strategies, and digital marketing tips. For any types of guest posting services, contact us on reblogit.webmail[at]gmail.com.