Asp.net is one of the superlative platforms used to develop impeccable developments with top-class security and features. To name just a few of those features, they are language interoperability, Action filters, container support, Globalization, Localization, Rich development environment, etc.that makes it even stronger.
Despite these, your project success would highly depend on Asp.Net developers you hire for your business project.However today, in this article we will learn about the development of API in .NET with GraphQL. What does GraphQL mean? How to configure GraphQL within the .NET application. We will deep dive into the concept briefly.
Why GraphQL?
APIs allow programs to talk to each other. At this point, the dominant design model is REST. Although it is known for building sustainable APIs, it has issues that come with today’s apps, particularly with mobile app consumers’ rise. Requests made with REST require numerous HTTP endpoints that return fixed data structures that can easily cause excess data. Problems also occur with frequent updates to applications, as it is very difficult to know individual clients’ needs.
What is GraphQL?
GraphQL was created by Facebook in 2012 (publicly published and open source in 2015) for its mobile and web apps. It was conceived precisely for more flexibility and efficiency in client communication and server.
GraphQL has an “intelligent” termination point that can take complex operations and return only the data the client needs. It is an open-source framework for developing and deploying fewer GraphQL backends. GraphQL is a data search language for APIs and runtimes to respond to these requests with your existing data (GraphQL.org).
GraphQL was created to solve the problems of the APIs of the time, especially with excessive retrieval. This was caused by the basic query structure, causing a series of queries with excessive data. This is particularly an issue with mobile applications thatare used with devices and network conditions that can’t handle huge 9 quantities of data and payloads. Today, it is maintained by a vast community around the world comprised of both businesses and individuals.
Advantages
When using GraphQL, the complexity of the client decreases drastically because it no longer needs to understand the different HTTP verbs, endpoints, and query paths. Because the server can only reply to client requests in a specific format, the client no longer needs to understand the different response codes of the API.
Another issue that GraphQL resolves is overheating data. When using GraphQL, only data that is relevant to the client is returned as a response to a request. On the other hand, GraphQL also resolves the data sub-extraction problem which is also known as the N+1 problem. For example, with REST services, the customer may need to make multiple inquiries to obtain relevant data, for example for a product, and then for product details.
Since the client and server are straightforward, applications can be developed iteratively, and changes on clients do not require changes on the server in most cases. Server-side code can be independently controlled, and data drives that are never used by clients can be deprecated without affecting clients.
GraphQL also supports data updates through such operations. mutations. Later in this article, we will see how we should not write code for various versions different requests, and versions of the mutations and that the entire process is fairly simple.
GraphQL Server
We are going to use the .Net WebAPI model to create our GraphQL API. Here are the steps you should follow when creating our API. Details of requests and deployments will be covered once we have provisioned our API. Install GraphQL packages into the application.
GraphQL is a query language for APIs and an execution environment to respond to these requests with your existing data. GraphQL provides a comprehensive and comprehensible description of your API data, empowers clients to request exactlywhat they need GraphQL is a query language for APIs and an execution environment to respond to these requests with your existing data.
Start by creating a .Net web API project. To integrate the graph API client, we need to install two NuGet packets:
- Client
- Client.Serializer.Newtonsoft
To get the GraphqlHttpClient, we can build the GraphqlClientBase class, which looks like.
public abstract class GraphqlClientBase
{
public readonly GraphQLHttpClient _graphQLHttpClient ;
public GraphqlClientBase()
{
if (_graphQLHttpClient == null)
{
_graphQLHttpClient = GetGraphQlApiClient();
}
}
public GraphQLHttpClient GetGraphQlApiClient()
{
var endpoint = “https://testproject1.hasura.app/v1/graphql”;
var httpClientOption = new GraphQLHttpClientOptions
{
EndPoint = new Uri(endpoint)
};
return new GraphQLHttpClient (httpClientOption, new NewtonsoftJsonSerializer ());
}
}
This class can be inherited where the HttpClient is required.
The NewtonsoftJsonSerializer class can be found inGraphQL.Client.Serializer .newtonsoftlibrary.
There are three basic concepts within GraphQL which are.
- Query – retrieves data from its corresponding data source.
- Move – update/change data.
- Subscribe – To view/monitor data.
We focus on how to make queries and transfers to GraphQL via GraphqlApiClient, To do this, we can create an ITransferService service agreement.
public interface ITransferService
{
Task<IEnumerable<TransferDto>> GetTransfers();
}
The actual implementation is given in the following section.
public class TransferService : GraphqlClientBase, ITransferService
{
public async Task<IEnumerable<TransferDto>> GetTransfers()
{
var query = @”query MyQuery {
Transfer {
Id
destinationTenantId
originTenantId
originalManuscriptId
title
}
}”;
var request = new GraphQLRequest(query);
var response = await _graphQLHttpClient.SendQueryAsync<TransferQueryResponse>(request);
return response.Data.Transfer;
}
}
Where TransferDto and TransferQueryResponse are categories of models.
public class TransferQueryResponse
{
public IEnumerable<TransferDto> Transfer { get; set; }
}
public class TransferDto
{
public int Id { get; set; }
public int OriginalManuscriptId { get; set; }
public string Title { get; set; }
public string OriginTenantId { get; set; }
public string DestinationTenantId { get; set; }
}
The GraphqlHttpClient class has the SendQueryAsync method which is responsible for the recovery of data(request) from the GraphQL database.
Similarly,GraphqlHttpClient class have method for mutation (modify data) is SendMutationAsync.
The GraphQLRequest object stores the request and variables for the corresponding graphicQL request/transfer.
To call this way GetTransfers(), we can create a TransferController controller.
[Route(“api/[controller]”)]
[ApiController]
public class TransferController : ControllerBase
{
private readonly ITransferService _transferService;
public TransferController (ITransferService transferService)
{
_transferService = transferService;
}
[HttpGet, Route(“get-all”)]
public async Task<IActionResult> GetTransfer()
{
try
{
var transfers = await _transferService.GetTransfers();
return Ok(transfers.OrderBy(s => s.Id));
}
catch (Exception ex)
{
throw ex;
}
}
}
We must also record dependencies in the ConfigureService method in Startup.cs.
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped<ITransferService, TransferService>(); //add this line
services.AddControllers();
}
Back after doing all this code, we can run the project by pressing f5 or Debug=> Start Debugging in the menu bar when we call the https://localhost: {your_port}/API/transfer/get-all.
We will obtain all data through graphqsslapi.
Conclusion
After going through the blog, you will get to know about how to prepare the ASP.NET Client application for consumption of the GraphQL application. And also, be knowing how to create requests and changes from a consuming application.
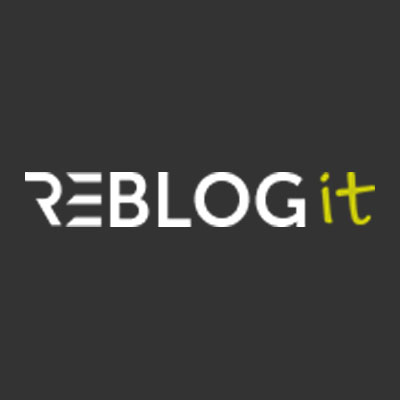
Reblog It collaborates closely with clients to develop tailored guest posting strategies that align with their unique goals and target audiences. Their commitment to delivering high-quality, niche-specific content ensures that each guest post not only meets but exceeds the expectations of both clients and the hosting platforms. Connect with us on social media for the latest updates on guest posting trends, outreach strategies, and digital marketing tips. For any types of guest posting services, contact us on reblogit.webmail[at]gmail.com.